Share your love
Execute Shell Commands in Python with Examples
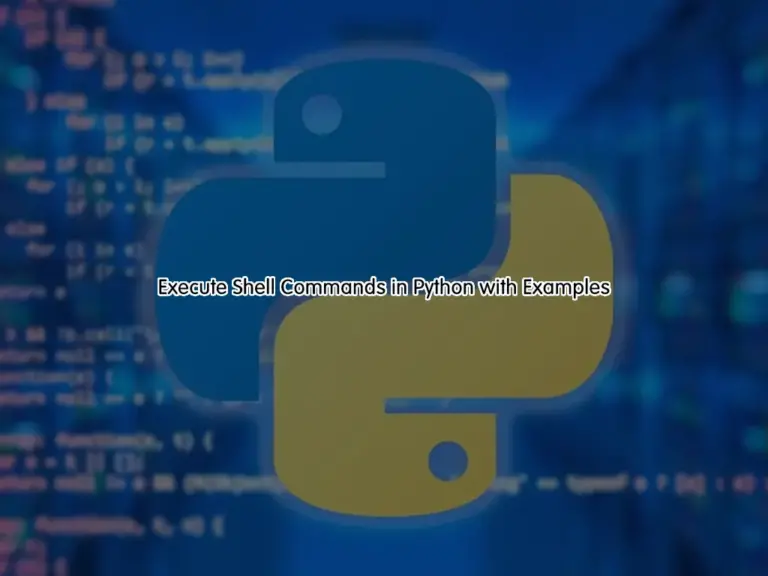
In this guide, we want to teach you to Execute Shell Commands in Python with Examples. As you know, Shell is a command interpreter for Linux systems. Shell commands can be used to perform the tasks. You may just know that shell commands can only run in a bash, but you can run them in a Python environment too. Here we will show you some examples of executing Shell commands in Python.
What is a Python shell command?
The Python Shell is the interpreter that executes your Python programs, other pieces of Python code, or simple commands.
How do you run a shell command in Python?
You can use different Python modules to run the Linux shell commands. In this guide, we try to discuss some of them and how you can use them. Follow the steps below to complete this guide.
Steps To Execute Shell Commands in Python with Examples
At this point, we want to show some various methods that you can Linux shell commands in Python. They are listed below:
- os.system()
- subprocess.run()
- subprocess.popen()
- os.popen()
Let’s get familiar with them and how to use them.
Step 1 – Use os.system Module in Python To Run Shell Commands
The os module can help you perform basic shell commands in Python including creating and removing a directory (folder), fetching its contents, changing and identifying the current directory, etc. From your Python file, you can use the following syntax to execute the shell commands:
import os
os.system('your desired shell command')
For example, we show you how to use the echo command in the above syntax:
# Importing os module
import os
# print message using os module
os.system('echo "How to execute shell commands in Python"')
This will print the message for you with the echo Linux command.
You can use any basic Linux shell commands in the os.system module. Another example for checking the timezone:
# Importing os module
import os
# Display the current timezone using the 'timedatectl' Linux command
os.system('timedatectl')
This will print the current timezone.
Step 2 – Use os.popen Module in Python To Execute Shell Commands
The os.popen method opens a pipe from a command. This pipe allows the command to send its output to another command. The output is an open file that can be accessed by other programs. For example:
import os
os.popen('pwd')
os.popen('pwd').read()
With this option, you can easily read the content of the object.
Step 3 – Use subprocess.run Module To Run Shell Commands
Another way that you can use to run shell commands in Python is by using the subprocess.run module. It is a great way that you can capture the output and errors.
For example:
import subprocess
subprocess.run('echo "how to use subprocess module"', shell=True )
When the parameter shell=True is used, the command is executed in a sub-shell. If you don’t use it, you will get an error.
If you don’t want to use any argument, you should use the command in the following way:
import subprocess
subprocess.run(['echo’, 'hello world’] )
Step 4 – Use subprocess.Popen Module to Run Shell Commands in Python
At this point, you can also use the subprocess.Popen module to execute the shell commands. The `subprocess.Popen` is a lower-level interface to running subprocesses, while subprocess.run is a higher-level wrapper around Popen that is intended to be more convenient to use.
For example:
import subprocess
subprocess.Popen(['ls -lh'], shell=True)
This will display the list of directories and files with sizes on the terminal.
To get more information, you can visit the Python Docs page.
Conclusion
At this point, you have learned to use various modules to run the Linux shell commands in Python with Examples. Hope you enjoy it. If you need any help, please comment for us.
Also, you may be interested in these articles:
Run a Python Script in a Linux Docker Container